Diving into Monero Development: Essential GitHub Libraries for Beginners
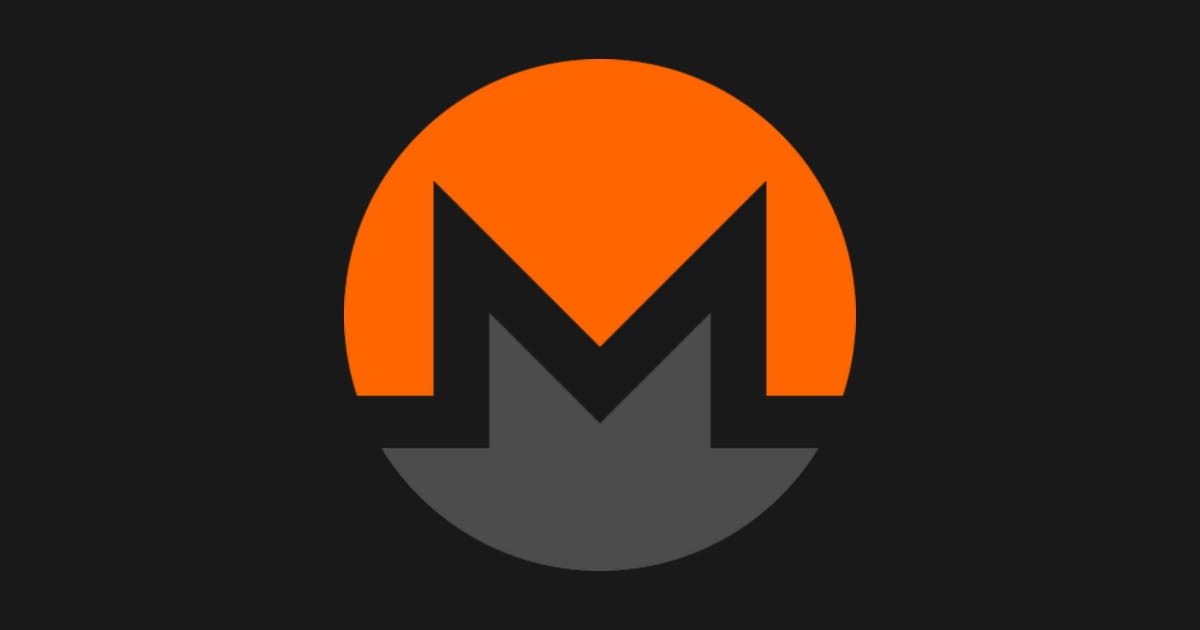
Monero, the privacy-focused cryptocurrency, stands as a testament to the power of open-source collaboration. If you're looking to contribute to this exciting project or build applications within the Monero ecosystem, understanding the core libraries used by developers is crucial. GitHub is the central hub for Monero development, and within its repositories lie a wealth of tools and libraries that power this digital currency.
This article will guide you through some essential GitHub libraries that Monero developers frequently use, focusing on three key areas: cryptographic operations, network communication, and tools for building and testing. We'll break down these complex topics into digestible pieces, perfect for beginners eager to start their Monero development journey.
1. The Foundation of Privacy: Cryptographic Libraries
At its heart, Monero's privacy features are built upon robust cryptography. Understanding and utilizing cryptographic libraries is paramount for any Monero developer. These libraries provide the fundamental building blocks for securing transactions, protecting user identities, and ensuring the overall integrity of the Monero network.
While Monero's core cryptography is largely implemented within its main codebase, understanding general cryptographic principles and using well-established libraries for related tasks is essential. Let's explore some key concepts and libraries relevant to cryptographic operations in the context of Monero development.
Understanding Cryptographic Primitives
Before diving into specific libraries, it's helpful to grasp some fundamental cryptographic primitives that are crucial for Monero and cryptocurrency in general:
- Hashing: Creating a fixed-size 'fingerprint' of data. Even a tiny change in the input data results in a drastically different hash. Used for data integrity and creating unique identifiers.
- Symmetric-key Encryption: Using the same key for both encryption and decryption. Efficient for encrypting large amounts of data.
- Asymmetric-key Encryption (Public-key Cryptography): Using key pairs (public and private keys). Public keys can be shared freely, while private keys must be kept secret. Used for secure communication and digital signatures.
- Digital Signatures: Using private keys to create a signature that can be verified by anyone with the corresponding public key, ensuring authenticity and non-repudiation.
Monero's core cryptography relies heavily on custom implementations of these primitives tailored for privacy, including Ring Signatures, Confidential Transactions, and Stealth Addresses. While you might not directly reimplement these from scratch as a beginner, understanding the underlying cryptographic concepts they employ is crucial.
Essential Libraries and Their Role
While Monero's core crypto is in-house, external libraries can still be valuable for auxiliary tasks or for understanding the broader cryptographic landscape. Here are some examples of libraries that might be encountered or are generally useful in cryptographic programming:
- OpenSSL: A widely used, mature cryptography library. OpenSSL provides a vast array of cryptographic algorithms and protocols. While perhaps more complex to use than
libsodium
for beginners, OpenSSL is a foundational library in many security-related projects and understanding its existence and capabilities is valuable.While Monero doesn't directly rely on OpenSSL for its core cryptographic operations, OpenSSL might be used in supporting infrastructure or tools. Understanding OpenSSL's command-line tools, for example, can be helpful for tasks like generating keys or certificates in related development scenarios. - Botan: Another robust C++ cryptography library, aiming for modern standards and ease of use. Botan offers a good balance of features and usability, and is another excellent choice for general cryptographic tasks.
libsodium: A modern, easy-to-use cryptography library. It provides a wide range of cryptographic primitives, including symmetric and asymmetric encryption, hashing, and digital signatures. While not directly used as Monero's core crypto library, libsodium
is a fantastic general-purpose crypto library to learn and might be used for related tooling or applications around Monero.Let's imagine you're building a tool to securely store user data related to their Monero wallet (though actual wallet security should always rely on official Monero software). You might use libsodium
to encrypt this data. Here's a simplified conceptual example in C++ (using libsodium
's C++ bindings):
#include <iostream>
#include <sodium.h>
#include <string>
#include <vector>
int main() {
if (sodium_init() == -1) {
std::cerr << "Sodium library initialization failed!" << std::endl;
return 1;
}
// Generate a secret key (in a real application, handle securely!)
unsigned char key[crypto_secretbox_KEYBYTES];
crypto_secretbox_keygen(key);
// Data to encrypt
std::string plaintext = "Sensitive Monero related data";
std::vector<unsigned char> ciphertext(plaintext.length() + crypto_secretbox_NONCEBYTES + crypto_secretbox_MACBYTES);
unsigned char nonce[crypto_secretbox_NONCEBYTES];
randombytes_buf(nonce, sizeof nonce);
// Encryption
crypto_secretbox_easy(&ciphertext[0], (const unsigned char*)plaintext.c_str(), plaintext.length(), nonce, key);
std::cout << "Encryption successful (conceptual example)." << std::endl;
// In a real application, you would store the nonce and ciphertext securely.
return 0;
}
Important Note: This is a simplified, conceptual example. Real-world cryptographic implementations require careful consideration of security best practices, key management, and potential vulnerabilities. Always consult with security experts when implementing cryptography in production systems.
Focus on Secure Coding Practices
Regardless of the specific cryptographic library you choose, secure coding practices are paramount when working with cryptography. This includes:
- Proper Key Management: Storing keys securely and avoiding hardcoding them in your applications.
- Nonce and Initialization Vector (IV) Handling: Using nonces and IVs correctly to ensure the security of encryption schemes.
- Avoiding Common Pitfalls: Being aware of common cryptographic vulnerabilities like padding oracle attacks or timing attacks.
- Staying Updated: Keeping your cryptographic libraries updated to patch any security vulnerabilities.
By understanding these fundamental cryptographic concepts and exploring libraries like libsodium
, you'll build a solid foundation for contributing to and developing within the Monero ecosystem. Remember to always prioritize security and consult with experts when dealing with sensitive cryptographic operations.
2. Connecting to the Network: Network Communication Libraries
Monero is a decentralized cryptocurrency, meaning it operates on a peer-to-peer (P2P) network. For Monero nodes and applications to function, they need to communicate effectively with other nodes on the network. This is where network communication libraries come into play.
These libraries provide the tools to establish connections, send and receive data, and manage network protocols. Understanding how Monero's network communication works and the libraries involved is crucial for building any application that interacts with the Monero blockchain or network.
Monero's Network Architecture
Monero utilizes a P2P network to propagate transactions and blocks. Nodes on the network connect to each other, forming a decentralized mesh. Key aspects of Monero's network communication include:
- Peer Discovery: Nodes need to find other nodes to connect to. This involves mechanisms like DNS seeding and peer exchange.
- Protocol Communication: Nodes communicate using specific protocols to exchange information about transactions, blocks, and network status.
- Data Serialization: Data needs to be converted into a format suitable for transmission over the network.
- Asynchronous Communication: Network operations are often asynchronous, meaning they don't block the main program execution while waiting for network responses.
Essential Libraries for Network Communication
Monero's core codebase is written in C++, and it leverages powerful C++ libraries for network communication. Here are some key libraries and concepts:
- Protocol Buffers (protobuf): A language-neutral, platform-neutral, extensible mechanism for serializing structured data. Monero uses Protocol Buffers to define the format of messages exchanged between nodes on the network. This ensures efficient and consistent data communication across different Monero implementations.When you send a transaction or request block data on the Monero network, the data is likely serialized using Protocol Buffers before being transmitted. Understanding Protocol Buffers is important for anyone working with Monero's network protocol. You can define message structures in
.proto
files and then use the protobuf compiler to generate code in various languages (including C++) to serialize and deserialize these messages. - libuv: Another high-performance, cross-platform asynchronous I/O library. While Monero primarily uses Boost.Asio, understanding libraries like
libuv
is beneficial as they represent common approaches to asynchronous networking and are used in other cryptocurrency projects and general software development.
Boost.Asio: A cross-platform C++ library for network and low-level I/O programming. Boost.Asio provides a robust and efficient framework for handling network connections, asynchronous operations, and protocol implementation. Monero heavily relies on Boost.Asio for its network layer.Boost.Asio allows developers to write code that can handle network events efficiently and non-blocking. Imagine you're building a simple Monero network monitoring tool. You would use Boost.Asio to connect to Monero nodes, send requests for network information, and receive responses.While a full Boost.Asio example is beyond the scope of this beginner article, here's a conceptual snippet demonstrating the idea of asynchronous socket connection (simplified and conceptual):
#include <boost/asio.hpp>
#include <iostream>
using boost::asio::ip::tcp;
int main() {
try {
boost::asio::io_context io_context;
tcp::resolver resolver(io_context);
tcp::resolver::results_type endpoints =
resolver.resolve("node.monerod.org", "18080"); // Example Monero node address (replace with actual)
tcp::socket socket(io_context);
boost::asio::async_connect(socket, endpoints,
[&](const boost::system::error_code& error, const tcp::endpoint& endpoint) {
if (!error) {
std::cout << "Successfully connected to Monero node: " << endpoint << std::endl;
// Now you can send and receive data on the socket
} else {
std::cerr << "Connection error: " << error.message() << std::endl;
}
});
io_context.run(); // Run the event loop to handle asynchronous operations
} catch (std::exception& e) {
std::cerr << "Exception: " << e.what() << std::endl;
}
return 0;
}
Conceptual Example Note: This is a highly simplified, conceptual example to illustrate the use of Boost.Asio for asynchronous network connection. Real-world Monero network communication involves complex protocols, data serialization, and error handling. This example is for educational purposes to demonstrate the basic idea of asynchronous networking.
Learning Network Programming Concepts
To effectively work with network communication libraries in Monero development, it's helpful to learn fundamental network programming concepts:
- Sockets: The basic building blocks for network communication. Understanding TCP and UDP sockets is essential.
- Client-Server and Peer-to-Peer Models: Knowing the differences between these network architectures.
- Network Protocols (TCP/IP, HTTP, etc.): Understanding how data is transmitted over networks.
- Asynchronous Programming: Grasping the concepts of callbacks, futures, and promises for handling non-blocking network operations.
- Data Serialization and Deserialization: Techniques for converting data into a transmittable format and back.
By exploring these network communication libraries and concepts, you'll be well-equipped to understand and contribute to Monero's network layer and build applications that interact with the Monero network.
3. Building and Ensuring Quality: Tools for Development and Testing
Developing robust and reliable Monero applications requires a strong set of tools for building, testing, and debugging. The Monero project emphasizes code quality and security, and various tools are used to ensure these standards are met.
This section will explore some essential tools and practices for building and testing Monero-related software. These tools help streamline the development process, catch errors early, and ensure the stability and security of the Monero ecosystem.
Build Systems and Automation
- CMake: A cross-platform build system generator. CMake is used to manage the build process for Monero's core software and many related projects. CMake allows developers to define the build process in a platform-independent way, making it easier to build Monero on various operating systems and architectures.CMake uses
CMakeLists.txt
files to describe the project structure, dependencies, and build rules. When you build Monero from source, you'll typically use CMake to generate the actual build files (e.g., Makefiles on Linux, Xcode projects on macOS, Visual Studio solutions on Windows) that are then used to compile and link the code. - Autotools (GNU Autotools): Another build system, historically used in many open-source projects. While Monero primarily uses CMake now, you might encounter Autotools in older projects or related tools. Autotools consists of tools like
autoconf
,automake
, andlibtool
to automate the build configuration process. - Continuous Integration (CI): Automated systems that build and test code changes whenever they are pushed to a repository. Monero utilizes CI systems like GitLab CI to automatically build and test code contributions, ensuring code quality and catching integration issues early. CI is crucial for collaborative development and maintaining a stable codebase.
Testing Frameworks and Methodologies
Rigorous testing is essential for cryptocurrency software, given the high stakes involved. Monero development employs various testing methodologies and frameworks:
- Integration Testing: Testing how different components or modules of the system interact with each other. Integration tests verify that the system works correctly when different parts are combined.
- System Testing: Testing the entire system as a whole, simulating real-world scenarios. System tests might involve running a local Monero network (like Statenet or Testnet) and testing various functionalities like transaction sending, block synchronization, and wallet operations.
- Regression Testing: Running tests after code changes to ensure that new changes haven't introduced regressions (broken previously working functionality). CI systems often automatically run regression tests.
- Fuzzing: A testing technique that involves feeding a program with invalid, unexpected, or random input data to try and find bugs or vulnerabilities. Fuzzing is particularly important for security-sensitive software like Monero.
Unit Testing: Testing individual components or functions of the code in isolation. Unit tests verify that small pieces of code work as expected. Frameworks like Google Test (gtest) are commonly used for writing unit tests in C++.
#include "gtest/gtest.h"
#include "your_monero_component.h" // Example: Replace with actual component
TEST(YourComponentTest, ExampleTest) {
YourMoneroComponent component;
int result = component.someFunction(5);
ASSERT_EQ(result, 10); // Example assertion (replace with actual test)
}
This is a very basic conceptual example of a Google Test unit test. In real Monero development, unit tests would be much more comprehensive and cover various aspects of the code.
Debugging and Analysis Tools
- Debuggers (gdb, lldb): Essential tools for stepping through code, inspecting variables, and understanding program execution flow when debugging issues.
gdb
(GNU Debugger) andlldb
(LLVM Debugger) are common debuggers for C++ development on Linux and macOS respectively. - Profilers (perf, valgrind): Tools for analyzing program performance and identifying performance bottlenecks. Profilers can help optimize code for speed and efficiency.
perf
is a Linux profiling tool, andvalgrind
is a suite of tools for memory debugging, profiling, and more, available on various platforms. - Static Analysis Tools (clang-tidy, cppcheck): Tools that analyze code without actually running it to detect potential bugs, style violations, and security vulnerabilities. Static analysis tools can help improve code quality and catch issues that might be missed by manual code review.
GitHub and Collaboration
GitHub itself is a crucial tool for Monero development, providing:
- Version Control (Git): For managing code changes, tracking history, and collaborating effectively.
- Issue Tracking: For reporting and managing bugs, feature requests, and tasks.
- Pull Requests: For proposing code changes and facilitating code review.
- Code Review: A critical process for ensuring code quality and security.
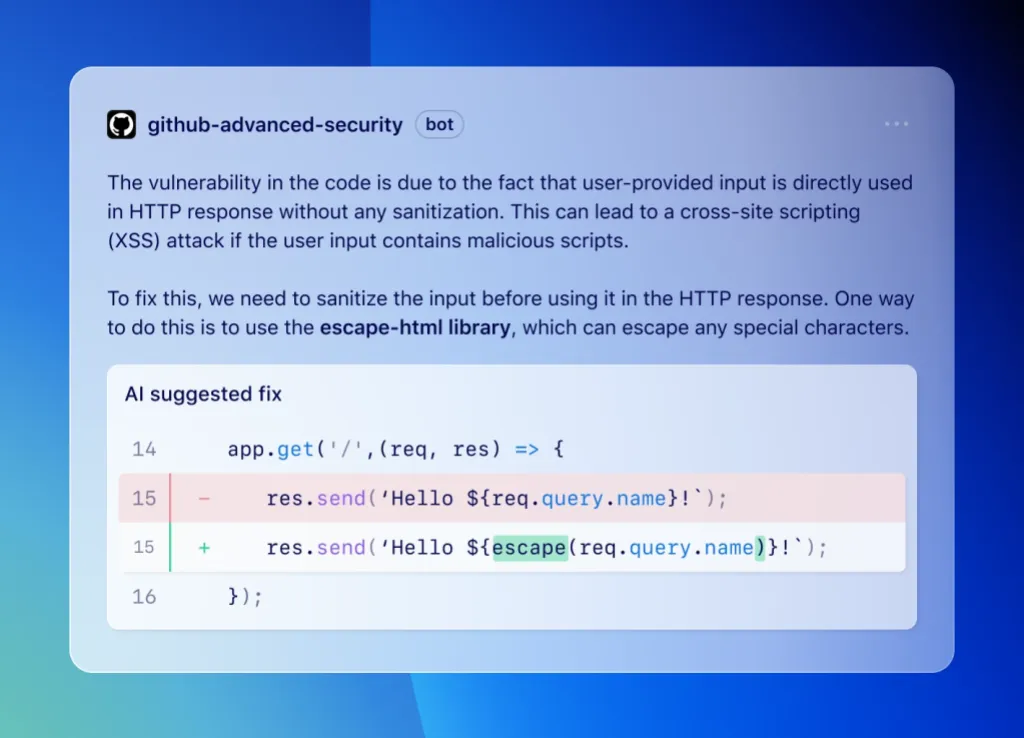
Image demonstrating GitHub code, highlighting code review or collaboration aspects.
By utilizing these build systems, testing frameworks, debugging tools, and leveraging the collaborative power of GitHub, Monero developers create and maintain a robust, secure, and high-quality cryptocurrency. As a beginner, familiarizing yourself with these tools and practices will greatly enhance your ability to contribute to the Monero project and build your own Monero-related applications.
Video: Monero Wallet GUI Statenet Setup - relevant to testing and development environment setup.
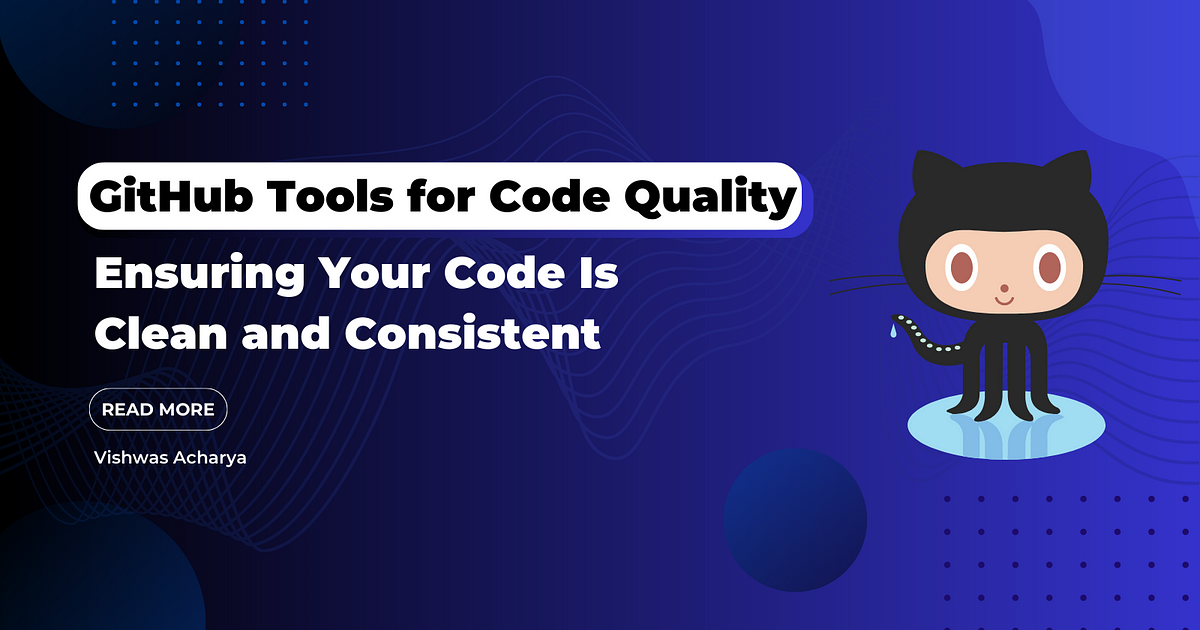
Image emphasizing code quality, perhaps showing static analysis output or testing metrics.
Conclusion: Your Journey into Monero Development
This article has provided a beginner-friendly overview of essential GitHub libraries and tools used in Monero development. From the cryptographic foundations that ensure privacy, to the network communication libraries that power the P2P network, and the build and testing tools that guarantee quality, these libraries are the bedrock of the Monero ecosystem.
As you embark on your Monero development journey, exploring these libraries and concepts further will be invaluable. Dive into the Monero GitHub repositories, experiment with code examples, and engage with the Monero developer community. The world of Monero development is rich and rewarding, and by understanding these fundamental tools, you'll be well-prepared to contribute to this groundbreaking cryptocurrency. Remember that continuous learning and a focus on security are key to success in this exciting field.